Overview
This unit introduces object-oriented software design and implementation, including the use of complex data structures and algorithms.You will learn data structures, algorithms, and both theoretical and practical aspects of software engineering. The theory will focus on software processes, requirements engineering, modelling, architectural design, design patterns, software development methodology, testing, and quality assurance. You will also be introduced to the principles of software reuse, and development code management. You will develop multi-tiered software application consisting of presentation, application, and data persistence tiers. You will also learn test-driven software application development using appropriate tools, thus building solid foundations for software development.
Details
Pre-requisites or Co-requisites
Pre-requisites: (COIT11134 and COIT11237) OR (COIT11134 and COIT12167).
Important note: Students enrolled in a subsequent unit who failed their pre-requisite unit, should drop the subsequent unit before the census date or within 10 working days of Fail grade notification. Students who do not drop the unit in this timeframe cannot later drop the unit without academic and financial liability. See details in the Assessment Policy and Procedure (Higher Education Coursework).
Offerings For Term 1 - 2025
Attendance Requirements
All on-campus students are expected to attend scheduled classes - in some units, these classes are identified as a mandatory (pass/fail) component and attendance is compulsory. International students, on a student visa, must maintain a full time study load and meet both attendance and academic progress requirements in each study period (satisfactory attendance for International students is defined as maintaining at least an 80% attendance record).
Recommended Student Time Commitment
Each 6-credit Undergraduate unit at CQUniversity requires an overall time commitment of an average of 12.5 hours of study per week, making a total of 150 hours for the unit.
Class Timetable
Assessment Overview
Assessment Grading
This is a graded unit: your overall grade will be calculated from the marks or grades for each assessment task, based on the relative weightings shown in the table above. You must obtain an overall mark for the unit of at least 50%, or an overall grade of 'pass' in order to pass the unit. If any 'pass/fail' tasks are shown in the table above they must also be completed successfully ('pass' grade). You must also meet any minimum mark requirements specified for a particular assessment task, as detailed in the 'assessment task' section (note that in some instances, the minimum mark for a task may be greater than 50%). Consult the University's Grades and Results Policy for more details of interim results and final grades.
All University policies are available on the CQUniversity Policy site.
You may wish to view these policies:
- Grades and Results Policy
- Assessment Policy and Procedure (Higher Education Coursework)
- Review of Grade Procedure
- Student Academic Integrity Policy and Procedure
- Monitoring Academic Progress (MAP) Policy and Procedure - Domestic Students
- Monitoring Academic Progress (MAP) Policy and Procedure - International Students
- Student Refund and Credit Balance Policy and Procedure
- Student Feedback - Compliments and Complaints Policy and Procedure
- Information and Communications Technology Acceptable Use Policy and Procedure
This list is not an exhaustive list of all University policies. The full list of University policies are available on the CQUniversity Policy site.
Feedback, Recommendations and Responses
Every unit is reviewed for enhancement each year. At the most recent review, the following staff and student feedback items were identified and recommendations were made.
Feedback from 2024 Unit Teaching Comments Report
Assignment 2 should be a group assessment because Assessment 3 builds off of it. It would make more sense if groups designed the diagram together, then proceeded to code the program using the diagram from A2.
Group work can be incorporated into students' assessments through design and code walkthroughs conducted after each student has completed individual attempts at design, coding, and testing.
Feedback from 2024 Unit Teaching Comments Report
The availability of the recorded lectures and tutorials was delayed a little each week, so it felt I was constantly trying to catch up on weekly materials.
The Echo360 system currently processes recorded videos with a slight delay, likely due to an overload of simultaneous tasks. Starting in Term 2 of 2024, Echo360 will expedite video processing, and by next year, this issue should no longer be a concern.
Feedback from Self-reflection
The Assessment item 3 (project) should add more advanced security requirements.
We will enhance security by requiring passwords to be encrypted in the database table, and access to the system will necessitate answers to the built-in security questions.
- Create a software requirements specification in accordance with the principles of requirements engineering
- Apply modelling techniques to document architectural and system models as per the requirements specification
- Use complex data structures and algorithms in software application development
- Design and implement a multi-tiered software application consisting of presentation, application and data persistence tiers
- Conduct test-driven development, validation, verification testing, software project testing, and design walkthroughs in small teams.
Australian Computer Society (ACS) recognises the Skills Framework for the Information Age (SFIA). SFIA is a widely used and consistent definition of ICT skills. SFIA is increasingly being used when developing job descriptions and role profiles. ACS members can use the tool MySFIA to build a skills profile at
https://www.acs.org.au/professionalrecognition/mysfia-b2c.html.
This unit contributes to the following workplace skills as defined by SFIA 8. The SFIA code is included:
- Requirements Definition and management (REQM)
- User Experience Analysis (UNAN)
- Software Design (SWDN)
- System Integration and Build (SINT)
- Programming/Software Development (PROG)
- Database Design (DBDS)
- Testing (TEST)
- Quality Assurance(QUAS)
- Quality Management (QUMG)
Alignment of Assessment Tasks to Learning Outcomes
Assessment Tasks | Learning Outcomes | ||||
---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | |
1 - Practical and Written Assessment - 25% | |||||
2 - Practical and Written Assessment - 30% | |||||
3 - Project (applied) - 45% |
Alignment of Graduate Attributes to Learning Outcomes
Graduate Attributes | Learning Outcomes | ||||
---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | |
1 - Communication | |||||
2 - Problem Solving | |||||
3 - Critical Thinking | |||||
4 - Information Literacy | |||||
5 - Team Work | |||||
6 - Information Technology Competence | |||||
7 - Cross Cultural Competence | |||||
8 - Ethical practice | |||||
9 - Social Innovation | |||||
10 - Aboriginal and Torres Strait Islander Cultures |
Textbooks
Engineering Software Products: An Introduction to Modern Software Engineering
Edition: 1st (2019)
Authors: Ian Sommerville
Pearson Higher Ed
USA
ISBN: 9780135210642
Binding: Paperback
Java How to Program (EarlyObjects, Global Edition)
Edition: 11th (2018)
Authors: Paul Deitel & Harvey Deitel
Pearson
USA
ISBN: 978-1292223858
Binding: eBook
IT Resources
- CQUniversity Student Email
- Internet
- Unit Website (Moodle)
- Violet Uml editor 2.1.0 or later
- JDK 21 (available from https://www.oracle.com/java/technologies/downloads or https://jdk.java.net/21/)
- JavaFX (downloaded in NetBeans when use JavaFX Maven archetype)
- SceneBuilder 21 (or higher) (available from https://gluonhq.com/products/scene-builder, current version 21.0)
- Apache NetBeans IDE 20 (https://netbeans.apache.org/download/index.html)
- MySQL Community Server 8.0.29 or higher
All submissions for this unit must use the referencing style: Harvard (author-date)
For further information, see the Assessment Tasks.
j.jarvis@cqu.edu.au
Module/Topic
Generic Collections; Selection Sort
Chapter
Deitel & Deitel, (textbook) Chapter 16 and Section 19.6.1
Events and Submissions/Topic
Install and test software (Test by developing a simple JavaFX application using SceneBuilder).
Tutorial classes commence in week 1. Make sure that you register for a tutorial class.
Module/Topic
Testing Part 1; JUnit testing; Regular Expressions
Chapter
Sommerville, Engineering Software Products (textbook) chapter 9; CQU content; Deitel & Deitel (textbook), Section 14.7
Events and Submissions/Topic
Module/Topic
Java Database Application Development (JDBC)
Chapter
Deitel & Deitel (textbook) Chapter 24; CQU content
Events and Submissions/Topic
Assessment 1 (Programming Assignment )- Phase 1 (GUI) - due Wednesday 9:00am (AEST). Note the completed assignment (all phases) is due in week 6)
Tutorial - Assessment 2 Part A1- due in week 3 tutorial class (Assessment item 2 / Tutorial assessment submissions commence: this work is to be developed and submitted in weekly tutorial classes from week 3 -12)
In-class groups allocated in tutorial classes this week. Do NOT change your tutorial class after you are assigned to your group.
Module/Topic
Software Processes
Chapter
Sommerville, Software Engineering (reference book): Extracts from chapters 1-5; Sommerville, Engineering Software Products (textbook), chapter 1; CQU content
Events and Submissions/Topic
Tutorial - Assessment 2 Part A2- due in week 4 tutorial class
Module/Topic
Software Architecture
Chapter
Sommerville, Engineering Software Products (textbook), chapter 4; Sommerville, Software Engineering (reference book), chapter 6; CQU content
Events and Submissions/Topic
Tutorial - Assessment 2 Part A3- due in week 5 tutorial class. Includes group work.
Module/Topic
Chapter
Events and Submissions/Topic
Check that you have no catch-up classes scheduled this week
Module/Topic
Software Design
Chapter
CQU content
Events and Submissions/Topic
Tutorial - Assessment 2 Part A4- due in week 6 tutorial class. Includes group work.
Assessment 1 Due: Week 6 Monday (21 Apr 2025) 9:00 am AEST
Module/Topic
Reliable Programming
Chapter
Sommerville, Engineering Software Products (textbook), chapter 8; CQU content
Events and Submissions/Topic
Tutorial - Assessment 2 Part A5- due in week 7 tutorial class. Includes group work.
Module/Topic
Security and Privacy; Testing Part 2
Chapter
Sommerville, Engineering Software Products (textbook), chapter 7 and 9; CQU content
Events and Submissions/Topic
Tutorial - Assessment 2 Part A6- due in week 8 tutorial class
Assessment 2: Part B (Tutorial group work - case study group report) - due Friday 5:00pm (AEST)
Module/Topic
Recursion
Chapter
Deitel & Deitel (textbook) chapter 18
Events and Submissions/Topic
Tutorial - Assessment 2 Part A7- due in week 9 tutorial class
Assessment 3 Part A Phase 2 - (Applied Project - Programming Assignment) - due Wednesday 9:00am (AEST). Note the completed assignment (all phases) is due in week 12)
Module/Topic
Custom Data Structures
Chapter
Deitel & Deitel (textbook) chapter 21
Events and Submissions/Topic
Tutorial - Assessment 2 Part A8- due in week 10 tutorial class
Module/Topic
DevOps and Code Management
Chapter
Sommerville, Engineering Software Products (textbook), chapter 10; CQU content
Events and Submissions/Topic
Tutorial - Assessment 2 Part A9- due in week 11 tutorial class
Module/Topic
Algorithmic Efficiency
Chapter
Deitel & Deitel (textbook) chapter 19 (sections 19.1-19.5); CQU content
Events and Submissions/Topic
Tutorial - Assessment 2 Part A10- due in week 12 tutorial class
Assessment 3 Part A - (Applied Project - Programming Assignment) - complete assignment due Friday 11:30pm (AEST)
Assessment 3 Part B - in class quiz (held during the week 12 tutorial class)
Module/Topic
Chapter
Events and Submissions/Topic
Module/Topic
Chapter
Events and Submissions/Topic
Unit Coordinator: Dr Jacqueline Jarvis
Senior Lecturer, CQU Brisbane Campus
P +61 7 3023 4238
E j.jarvis@cqu.edu.au
1 Practical and Written Assessment
This is an individual assignment.
In this assignment, you will use the key constructs and concepts introduced in Weeks 1- 2 to develop and unit test a software application that employs a Graphical User Interface (a JavaFX GUI) and uses complex data structures and algorithms. You will be required to provide an acceptance test plan and the results of those tests. Further details are provided in the detailed Assignment 1 specification available on the Moodle unit website.
Note that:
- A separate phase 1 submission is required early in the development period for this assignment.
- All the phases are described in the detailed specification on the unit website. You are required to keep backups of all phases. You must submit both phase 1 (early in the development) and your completed project. There is a submission area for phase 1 and a separate submission area for the final submission. The due dates and times are available in the submission areas.
- You may be asked to demonstrate your understanding of the assignment to the unit coordinator before marks can be awarded.
- Re-attempts are not allowed for the final (week 6) submission of this assignment.
Week 6 Monday (21 Apr 2025) 9:00 am AEST
Note that Phase 1 is due Wednesday at 9:00am (AEST) in week 3. The final submission is due on Monday of week 6.
Two weeks after the assignment is submitted
This is an individual assignment and contributes to 25% of the total marks. This assignment will be assessed according to the following criteria:
- Design and Implementation (and functionality achieved)
- Language use including correct application of classes, data structures, algorithms, and programming good practices.
- Documentation
- Testing
- Use complex data structures and algorithms in software application development
2 Practical and Written Assessment
This assessment item will include both individual and group work. It has two parts, part A and part B described below.
In this assessment item you will be required to demonstrate your ability to apply the principles of requirement engineering by eliciting the functional and non-functional requirements, documenting requirements specification, modelling the system, and designing the system architecture and implementation.
In addition, you will be required to demonstrate an understanding of key topics covered each week.
Note: Re-attempts are not allowed for this assessment item. (See conditions below)
Part A: Tutorial work (20%)
This part of the assessment is to be developed and submitted as part of your weekly tutorial sessions. It consists of a series of 10 tutorial submissions to be developed and submitted in the tutorial class in weeks 3-12 (inclusive). For on-campus students this work is due in the weekly tutorial, NOT on the due date shown in Moodle. Moodle cannot have multiple due dates to cater for different tutorial times so the date specified in Moodle is to allow for tutorials held at the end of the week or on weekends. For on-campus students no marks will be awarded for work submitted outside the tutorial class or for late submissions unless there are special circumstances and the alternative submission is approved by the unit coordinator. If you have special circumstances that prevent you from attending a specific class, please contact your tutor and unit coordinator as soon as you are aware of the issue.
Some of the tutorial work will be individual and some will involve group work. You will be allocated your group in week 3. Some group work will be started in the tutorial class, but the group members will be required to finish work outside the class. It is important that all members of the group contribute equally to the group work. If any members of the group are not contributing to the work please discuss this with your tutor as soon as you are aware of the issue. It will be important to prepare for your tutorial by attending the lectures and reading the required materials prior to the tutorial class.
On-campus students
- This assessment item must be developed and submitted in your weekly tutorial as and when you are directed to do so by your tutor.
- Marks may be deducted if your tutor is not satisfied with your progress or understanding of the work or if you do not attend the whole tutorial. Make sure you are on time to class. Marks are not only awarded for the sample of work submitted during the class. Satisfactory participation in all the tutorial class activities is also required to be awarded marks for this assessment item.
Online/Distance Education students
- You will also be required to submit a specified sample of your weekly work by the due date specified by the coordinator. Late submissions will be awarded 0 marks unless you have been given approval for a late submission due to special circumstances.
- The unit coordinator or a member of the teaching team may make special arrangements to discuss aspects of the weekly work with you before marks can be awarded.
- You will also be required to work as part of a group for some of the tutorial work and to complete part B of this assessment item in your group.
All Students: Note that although you may only be required to submit a sample of your work in each tutorial, all the tutorial questions and exercises are important. Each week all the questions should be attempted and the answers checked. Any work not completed in the tutorial class should be completed outside class.
Each week's tutorial work is worth 2 marks. The total for this assessment item is 20%.
Part B: Group Case Study and Report (Design) (10%)
Weeks 5-7 of the tutorial work will involve group work to determine the requirements and the partial design specification for an application. The final output from this exercise is to be a report that documents the design process and partial design documentation for the application. The report is to be submitted as part B of this assessment item. Note that groups are also expected to work on this task outside the tutorial class. All members of the group are to contribute to the work and all group members are to submit a copy of the group's final report in Moodle. More details about the requirements and a template for the report document can be found on the unit website.
Part A: This part of the task commences with an "in-class" submission of work in week 3 and continues with "in-class" weekly submissions until the end of week 12 (i.e. 10 weekly submissions). On-campus students must complete and submit the scheduled work in their weekly tutorial class. Part B: The group report is due on Friday of week 8 at 5:00pm (AEST)
Part A: Tutorial marks will be awarded within a week after the date of submission. Part B: The marks for the group report will be returned 2 weeks after submission.
This assessment item involves both individual and group work. It contributes to 30% of the total marks. In this assessment item students are assessed on their ability to:
- Apply software design and development principles to the design of a 3-layered system.
- Complete appropriate documentation for requirements, system modelling, and design.
- Complete weekly tutorial questions and exercises that demonstrate an understanding of key topics covered each week.
- Create a software requirements specification in accordance with the principles of requirements engineering
- Apply modelling techniques to document architectural and system models as per the requirements specification
- Design and implement a multi-tiered software application consisting of presentation, application and data persistence tiers
3 Project (applied)
This assessment item consists of two parts - part A and part B. Re-attempts are not allowed for either part of this assessment item.
Both parts of this assessment item are individual tasks.
Part A: Programming assignment (30%)
In this part of the assessment task, you are required to develop and unit test a 3-layered software application. This application will have a presentation layer (a JavaFX GUI), an application layer and a database access layer. You will also be required to provide an acceptance test plan and the results of those tests. You will be using the topics learned in weeks 1- 9 in this assignment. Complete task details are in the Assignment 3 Part A specification document available on the Unit website.
Note that:
- A separate phase 2 submission is required early in the development period for this assignment. You are not required to submit phase 1 in this assessment item.
- All the phases are described in the detailed specification on the unit website. You are required to keep backups of all phases. You must submit both phase 2 (early in the development) and your completed project. There is a submission area for phase 2 and a separate submission area for the final submission. The due dates and times are available in the submission areas.
- You may be asked to demonstrate your understanding of the assignment to the unit coordinator before marks can be awarded.
- Re-attempts are not allowed for the final (week 12) submission of this assignment
Part B: In-class test (15%)
- On-campus students must sit the test in their tutorial class in week 12.
- Online students will be required to attend an online tutorial in week 12 to sit the test.
This in-class test will be held as part of your week 12 tutorial class. It will test content from weeks 1 to 11 inclusive. The week 12 tutorial class will also cover tutorial questions related to the week 12 content.
More details about the in-class test will be provided on the unit website during the term.
Part A: Phase 2 is due Wednesday of week 9. The complete assignment is due on Friday of week 12. Part B is an in-class test in the week 12 tutorial.
As this is the final assessment item, results cannot be released until certification date.
Part A:
In part A of this assessment item students are assessed on their ability to:
- Implement a 3-layered system that includes a graphical user interface (JavaFX GUI), application logic and database access objects.
- Rigorously test the software application.
Part B
Part B of this assessment item is an in-class test that assesses the student's knowledge and understanding of the content covered in weeks 1 - 11 inclusive.
- Create a software requirements specification in accordance with the principles of requirements engineering
- Apply modelling techniques to document architectural and system models as per the requirements specification
- Use complex data structures and algorithms in software application development
- Design and implement a multi-tiered software application consisting of presentation, application and data persistence tiers
- Conduct test-driven development, validation, verification testing, software project testing, and design walkthroughs in small teams.
As a CQUniversity student you are expected to act honestly in all aspects of your academic work.
Any assessable work undertaken or submitted for review or assessment must be your own work. Assessable work is any type of work you do to meet the assessment requirements in the unit, including draft work submitted for review and feedback and final work to be assessed.
When you use the ideas, words or data of others in your assessment, you must thoroughly and clearly acknowledge the source of this information by using the correct referencing style for your unit. Using others’ work without proper acknowledgement may be considered a form of intellectual dishonesty.
Participating honestly, respectfully, responsibly, and fairly in your university study ensures the CQUniversity qualification you earn will be valued as a true indication of your individual academic achievement and will continue to receive the respect and recognition it deserves.
As a student, you are responsible for reading and following CQUniversity’s policies, including the Student Academic Integrity Policy and Procedure. This policy sets out CQUniversity’s expectations of you to act with integrity, examples of academic integrity breaches to avoid, the processes used to address alleged breaches of academic integrity, and potential penalties.
What is a breach of academic integrity?
A breach of academic integrity includes but is not limited to plagiarism, self-plagiarism, collusion, cheating, contract cheating, and academic misconduct. The Student Academic Integrity Policy and Procedure defines what these terms mean and gives examples.
Why is academic integrity important?
A breach of academic integrity may result in one or more penalties, including suspension or even expulsion from the University. It can also have negative implications for student visas and future enrolment at CQUniversity or elsewhere. Students who engage in contract cheating also risk being blackmailed by contract cheating services.
Where can I get assistance?
For academic advice and guidance, the Academic Learning Centre (ALC) can support you in becoming confident in completing assessments with integrity and of high standard.
What can you do to act with integrity?
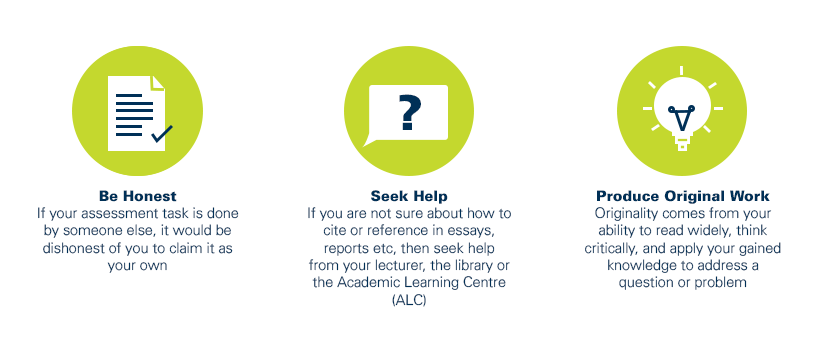